Understanding Hashing Tables: Concepts and Applications
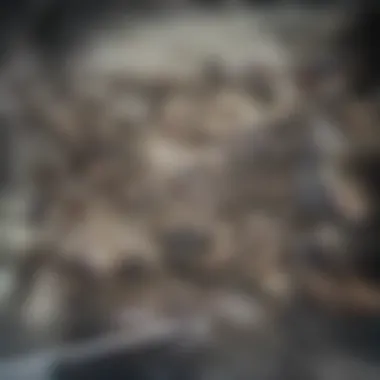
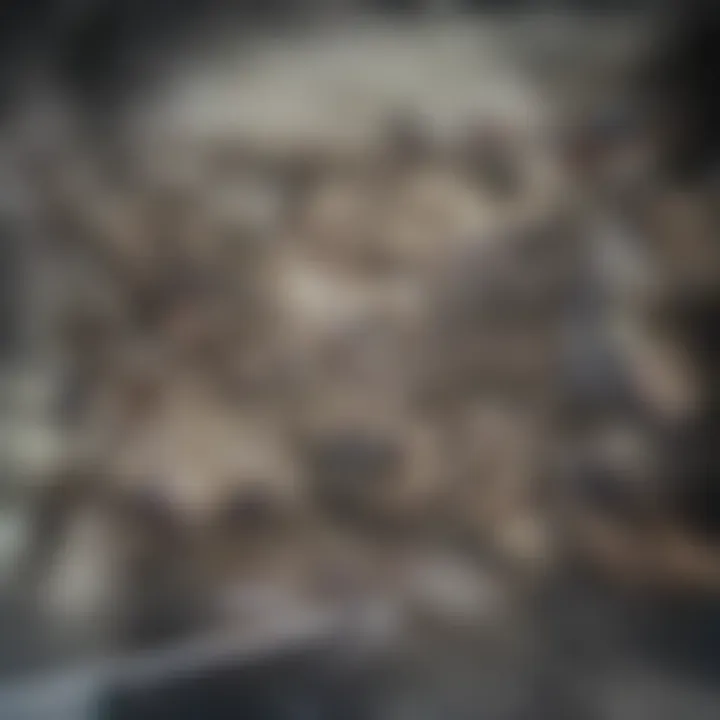
Intro
Hashing tables are essential data structures in computer science, commonly used for efficient data retrieval. They map keys to values, which allows for quick access to data. This article aims to explain the mechanics of hashing tables, their underlying principles, and the practical implications in various fields.
Understanding the basic concept of hashing is crucial, as it provides a foundation for exploring more complex applications. The efficiency of accessing elements is one of the notable advantages of using hashing tables. However, it is important to recognize the challenges involved, such as collision resolution and performance considerations. This article will give a thorough overview of these aspects.
Moreover, this exploration will highlight how hashing tables find use in numerous real-world applications. From database indexing to caches, their versatility makes them valuable in different scenarios.
As we delve into the key concepts surrounding hashing tables, we will look at the definitions of primary terms related to this topic, alongside associated theories. Let’s begin.
Key Concepts
Definition of Primary Terms
Before diving deeper, it is necessary to establish some fundamental terminology.
- Hash Function: A function that takes an input (or 'key') and produces a fixed-size string of bytes. The output is typically a hash code which is unique to each unique input.
- Hash Table: A data structure that pairs keys to values, using a hash function to calculate an index at which to store the value.
- Collision: This occurs when two different keys generate the same hash code, leading to potential issues in data retrieval.
- Load Factor: Represents the ratio of the number of entries in the table to the number of available slots. A higher load factor can lead to more collisions.
Related Concepts and Theories
Several theories enhance our understanding of hashing tables and their functions.
- Open Addressing: A collision resolution technique where, upon collision, an alternative index is sought within the hash table until an empty slot is found.
- Chaining: Another method to resolve collisions, where each slot in the hash table maintains a list of values that hash to the same index.
- Double Hashing: A more complex collision resolution that uses a second hash function to find the next available slot.
These concepts provide the groundwork for analyzing hashing tables. Understanding how they interact helps in grasping the applications and effectiveness of hashing tables in various software systems.
Future Directions
Gaps Identified in Current Research
While hashing tables are widely utilized, some research gaps remain. For instance, many current studies overlook the performance impact of specific hash functions under diverse loads and distributions.
Another gap exists in the evaluation of hashing techniques in distributed systems. How effective are traditional hashing methods in environments requiring rapid scalability? This remains an open inquiry.
Suggestions for Further Studies
Future research could focus on developing new hash functions that adapt to varying data types. Another direction might involve integrating machine learning techniques to optimize collision resolution.
Establishing benchmarks for different hashing strategies could also provide insights into their real-world efficacy.
Prologue to Hashing Tables
Hashing tables serve as a foundational structure in computer science. This section aims to illustrate their significance along with their utility in various applications, covering essential elements and vital considerations.
Definition and Purpose
A hashing table is a data structure that implements an associative array, allowing for efficient retrieval, insertion, and deletion of data. The core function is based on a hash function, which converts a given key into an integer index. This index determines the location where the corresponding value is stored. The primary purpose of using hashing tables is to maximize the efficiency of these operations, aiming for constant time complexity—O(1)—for ideal scenarios. This level of efficiency is particularly important in applications where quick access to data is needed, such as in databases and caching mechanisms.
The appeal of hashing tables lies in their ability to manage large datasets while maintaining performance. However, their effectiveness can vary based on the implementation of the hash function and how collisions are handled. Collisions occur when two keys map to the same index, necessitating strategies to resolve them effectively.
Historical Context
The concept of hashing has evolved significantly since its inception. In the early days of computing, data access was primarily linear, utilizing structures like arrays and linked lists. As data volumes grew, the limitations of these methods became clear, particularly when it came to speed and efficiency.
Around the 1950s, with the advancement of computer technology and algorithms, researchers began developing hashing techniques. One of the pioneering works was done by H.P. Luhn in 1953, who introduced the idea of using hashes for information retrieval.
Since then, the discipline has matured, with numerous improvements in hash algorithms and collision resolution techniques. Today, hashing tables are widely used in various areas, including software engineering, database management systems, and cryptographic functions. Their continued relevance underscores the importance of understanding their principles and applications in modern computational environments.
"Hashing tables transform the way we store and access data, providing the speed and efficiency needed in the data-driven world."
Fundamental Concepts
Understanding the fundamental concepts of hashing tables is essential for grasping their role in computer science and data management. The key components that make up hashing tables are influential in determining their efficiency and effectiveness in various applications. These concepts address core functionalities that underpin how data is managed, stored, and retrieved, which is critical for both theoretical understanding and practical implementation.
Key Components
Key components of hashing tables include the data structure itself, hash functions, and the method of handling collisions. The data structure allows for the storage and retrieval of information through associating keys with values. Hash functions play a vital role in mapping data to specific indices in the hash table. A well-designed hash function reduces the chances of collisions, which occur when two keys map to the same index.
- Data Structure: Typically, hashing tables utilize an array for storage.
- Hash Function: A function that takes an input (the key) and converts it to a specific index within the array. Its design directly affects performance.
- Collision Resolution: Techniques such as chaining or open addressing are used to manage cases where multiple keys hash to the same index.
Hash Functions Explained
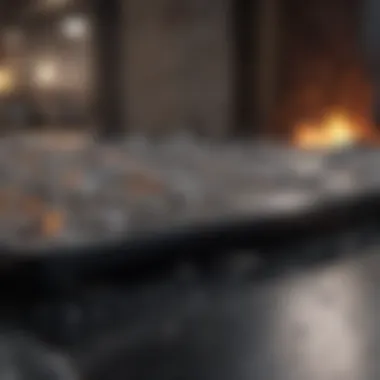
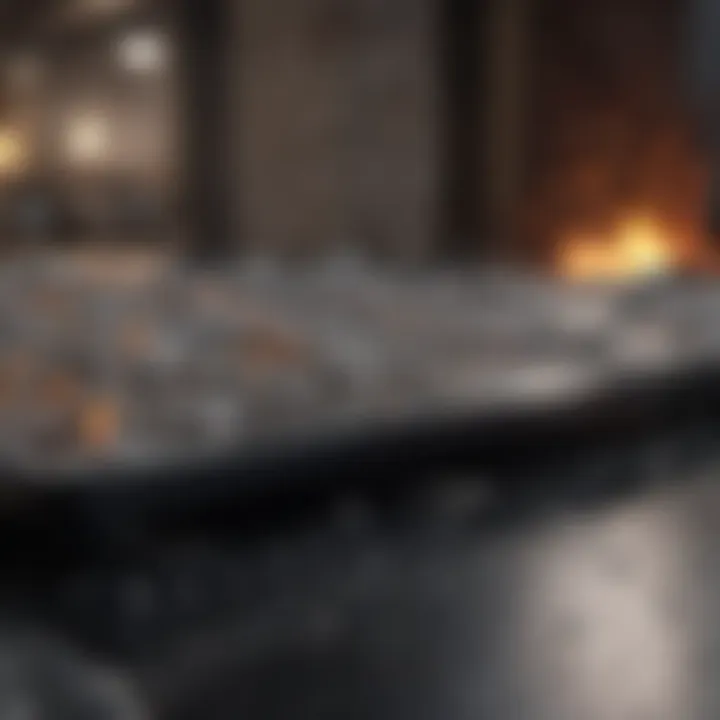
Hash functions are crucial in hashing tables. They provide a mechanism for converting keys into indices that can be used to access or store values. The effectiveness of a hash function can directly impact the performance and efficiency of the hashing table. A good hash function distributes keys uniformly across the available indices, minimizing the likelihood of collisions.
Factors to consider when designing a hash function include:
- Universality: A hash function should ideally work well across a wide range of possible inputs.
- Determinism: The output must be consistent; every input must yield the same output every time.
- Efficiency: It should be computationally simple and fast to compute the hash value.
Load Factor Considerations
The load factor is a metric that measures how full the hash table is. It is calculated as the ratio of the number of entries to the number of available slots. Understanding the load factor is key to managing a hashing table’s performance, as it influences retrieval and insertion times.
A higher load factor increases the probability of collisions and impacts performance negatively. Conversely, a load factor that is too low might lead to wasted space in memory. Thus, a balance must be struck. The ideal load factor is typically around 0.7 – 0.8 for many applications, but this can vary based on specific use cases and the collision resolution method employed.
"Maintaining an optimal load factor is essential for achieving balance between memory efficiency and operational speed."
In summary, grasping these fundamental concepts provides the groundwork for further exploration of hashing tables and their applications in various domains. Understanding the relationships between key components, hash functions, and load factors allows for better design choices and optimizations.
Hashing Algorithms
Hashing algorithms play a pivotal role in the functionality of hashing tables. They serve as the bridge between the input data (or keys) and their respective storage locations in the hash table. These algorithms translate input data into fixed-size numerical values, known as hash codes or hash values, that dictate where the corresponding data will reside in the table.
The significance of hashing algorithms lies in their ability to efficiently distribute data across a hash table. A well-designed hashing algorithm minimizes collisions—situations where two keys produce the same hash value—thereby maintaining optimal performance. Furthermore, the effectiveness of a hashing algorithm can directly influence both the speed and space efficiency of data retrieval processes. Hence, choosing an appropriate algorithm is fundamental for system performance.
Common Hashing Algorithms
Several hashing algorithms have become standard in computing, each possessing unique characteristics and use cases. They can be categorized into general hashing algorithms and specialized forms.
- Division Method: This simple technique divides the key by a prime number and uses the remainder as the index. It is straightforward but may suffer from clustering problems.
- Multiplication Method: Multiplication relies on multiplying the key by a fraction (often between 0 and 1) and then taking the fractional part. This method is sometimes preferred for its distribution qualities.
- Cryptographic Hash Functions: These are specialized hashing algorithms designed for security purposes, returning a fixed-size string regardless of input size.
- MurmurHash: Known for its performance, MurmurHash is widely used in non-cryptographic applications. It provides good distribution and efficiency.
Each algorithm brings distinct advantages and challenges, making the choice dependent on the specific requirements of the application, such as speed or security needs.
Cryptographic Hash Functions
Cryptographic hash functions are specialized algorithms essential for ensuring data integrity and security. They output a fixed-size hash value that is unique to the input data. The output of these functions must exhibit certain properties:
- Deterministic: The same input always yields the same output.
- Collision-Resistant: It should be infeasible to find two different inputs that yield the same output.
- Pre-image Resistance: Given a hash output, it should be hard to determine the original input.
- Small Changes Produce Drastic Changes: A minor alteration in input should lead to significant differences in the hash output.
Popular cryptographic hash functions include SHA-256 and MD5. SHA-256 is particularly used in various security protocols due to its robust nature and complexity in reversing the output to discover the original input.
These functions are crucial in applications such as digital signatures, data verification, and securing passwords. As cyber threats become more sophisticated, the importance of robust cryptographic hashing algorithms cannot be overstated.
Collision Handling Techniques
Collision handling is a crucial aspect of hashing tables. When two keys hash to the same index, a collision occurs. Properly managing these collisions is essential for maintaining performance and ensuring data integrity. Without effective collision handling, the efficiency of searching, inserting, or deleting operations can significantly decline.
Chaining Method
The chaining method resolves collisions by maintaining a list of all elements that hash to the same index. Each index in the hash table serves as a pointer to a linked list (or another data structure) that holds all the values sharing that index.
Benefits of Chaining
- Memory Efficiency: As new collisions occur, memory is allocated for each new entry without resizing the entire table.
- Simplicity: This method is straightforward to implement.
- Flexibility: Allows the hash table to handle an arbitrary number of entries without a significant reorganization.
Considerations
The primary downside is that the time complexity can degrade to linear if many keys hash to the same index, making the average retrieval time higher than desired. Nevertheless, appropriate hash functions typically help mitigate this issue.
Open Addressing
Open addressing, unlike chaining, finds a vacant slot within the hash table itself. When a collision occurs, the algorithm probes for the next available index, following a specific probing sequence.
Probing Techniques
- Linear Probing: Check the next slot sequentially.
- Quadratic Probing: Check the slots using a quadratic function based on the number of attempts.
- Random Probing: Randomly select slots based on a predefined range.
Benefits of Open Addressing
- Space Efficiency: More memory efficient since it utilizes fewer pointers.
- Cache Performance: It excels in scenarios with a low load factor, providing better locality of reference.
Considerations
Open addressing may lead to clustering, where groups of consecutive slots are filled, resulting in long search times. Therefore, managing the load factor is critical in open addressing.
Double Hashing
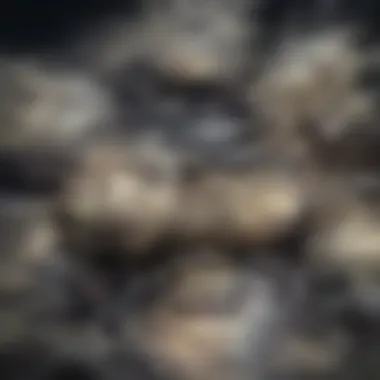
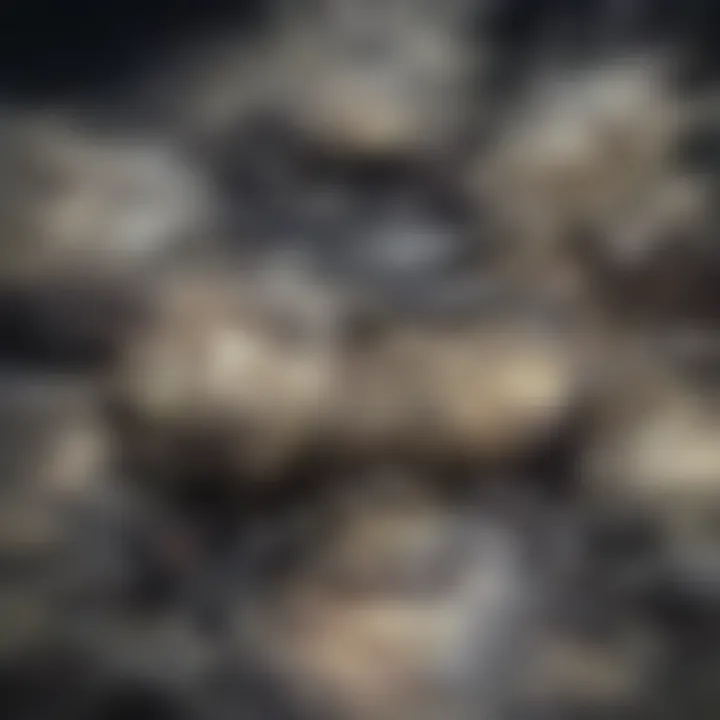
Double hashing is an advanced form of open addressing that uses a second hash function to determine the probing sequence when a collision occurs. It helps address the clustering issue associated with other probing methods.
How it Works
When a collision occurs at a given index, a second hash function calculates a jump distance. This jump modifies the probing sequence, reducing the chances of clustering.
Benefits of Double Hashing
- Reduced Clustering: By varying the probing steps, clustering is less likely, which often leads to better average search times.
- Effectiveness in High Load Factors: This method is useful even when the hash table has a higher load factor than other methods.
Considerations
The implementation of double hashing can be more complex due to the requirement of two hash functions. Additionally, care must be taken to ensure that the second hash function does not evaluate to zero, which would create an infinite loop in probing.
Effective collision handling methods are vital in maintaining the performance of hashing tables in practical applications.
Performance Analysis
Performance analysis is a crucial aspect for understanding the efficiency and effectiveness of hashing tables. It encompasses evaluations related to speed and memory utilization, both paramount to system performance. Employing architectures with inefficient hashing mechanics can lead to slow performance, frustrating users and developers alike. Thus, performance analysis provides insights into how hashing tables function under varying workloads and scales.
Time Complexity
Time complexity in hashing tables is generally expressed in terms of average case and worst-case scenarios. In the average case, when the hash function distributes entries uniformly, operations like insertion, deletion, and search execute in constant time, O(1). This efficiency stems from the direct addressing applied in the data structure. However, worst-case complexity arises due to potential collisions that may accumulate, leading to operations taking linear time, O(n), particularly when many entries map to the same index.
For instance, employing effective hash functions can significantly decrease the chances of collisions. A poor hash function may lead to clustering, where many keys hash to the same value. Therefore, it is vital to analyze hashing patterns and adjust the design of hash functions accordingly.
- Average Case: O(1)
- Worst Case: O(n)
Space Complexity
Space complexity of hashing tables refers to the amount of memory they require to store data. The overall space consumed can be considerable, especially when compared to other data structures like arrays or linked lists. The primary influence on space complexity is the load factor, defined as the ratio of the number of stored entries to the size of the array. A higher load factor usually denotes less empty space, which may lead to more collisions. Conversely, a lower load factor might result in unused memory.
Knowing the space complexities allows developers to optimize the tables depending on use cases. For example, dynamic resizing can be implemented, where the table expands or shrinks given changes in load factors. Ensuring that the table does not exceed a predetermined threshold allows for good performance, stability, and resource management. The complexity analysis helps in making informed decisions about when to resize and how to maintain efficient memory usage.
Applications of Hashing Tables
Hashing tables play a pivotal role in various domains of computing, showcasing their utility in numerous applications. They facilitate data organization and retrieval, a necessity in modern software systems. The benefits of hashing tables include improved performance, faster search times, and optimized resource usage. This section explores several specific applications where hashing tables are indispensable.
Database Management
In the world of database management, hashing tables serve as a foundational structure for indexing and quick query resolution. By utilizing a hash function, relational databases like PostgreSQL and MySQL can map unique keys to their corresponding records. This technique significantly accelerates search operations, often reducing the time complexity from linear to constant time under ideal conditions.
Moreover, hashing can aid in ensuring data integrity and consistency. Hash indexes can validate data retrievals against original stored values, enhancing security. It’s also useful for implementing unique constraints within tables, ensuring data entries are distinct and reliable.
Caching Mechanisms
Caching mechanisms leverage hashing tables to store frequently accessed data temporarily, thereby minimizing delays in data retrieval. For instance, web servers employ caching to hold responses for previous requests, thus reducing server load and network latency. Tools like Redis and Memcached utilize hashing strategies to optimize the storage and retrieval of key-value pairs.
The benefits in this context include reduced response times and lower bandwidth consumption. Caches, backed by efficient hashing, enable a smooth user experience by providing rapid access to data that would otherwise require extensive processing or database queries.
Data Integrity Verification
Data integrity is critical in data storage and transmission, and hashing tables excel in this area. Techniques like checksum algorithms utilize hashing to create a representation of data, which can be compared at both ends of data transfer. This ensures that the data remains unaltered during transmission.
Hashing provides a fast method to detect errors or unauthorized changes. For applications such as blockchain and secure communications, maintaining data integrity is non-negotiable. Hash functions like SHA-256 are widely implemented in such scenarios, providing secure verification processes.
In summary, hashing tables augment both the performance and security of data operations across numerous fields. Their applications range from optimizing database performance to ensuring data integrity in distributed systems.
Overall, the application of hashing tables extends far beyond mere storage. They help streamline processes, enhance data retrieval efficiency, and secure information in a fast-paced digital environment.
Case Studies
Case studies play a crucial role in understanding the practical implementation and effectiveness of hashing tables. These real-world examples demonstrate the tangible benefits and challenges associated with hashing techniques. By examining various case studies, readers can gain insights into how hashing is applied in diverse fields and learn about the outcomes of its applications. Such examples provide context and help illustrate theoretical concepts discussed in this article.
Hashing in Algorithm Optimization
Algorithm optimization is vital in enhancing the efficiency of software applications. Hashing tables serve as a powerful tool in this respect. For example, in searching and sorting operations, employing hashing techniques significantly reduces the time complexity compared to traditional methods.
When an algorithm leverages hashing, it can achieve average-case constant time complexity for lookups. This is a stark contrast to the linear time complexity often observed with arrays or linked lists. A prominent case is the implementation of hash tables in programming languages like Python and Java. They utilize built-in hash functions to optimize their data storage and retrieval mechanisms.
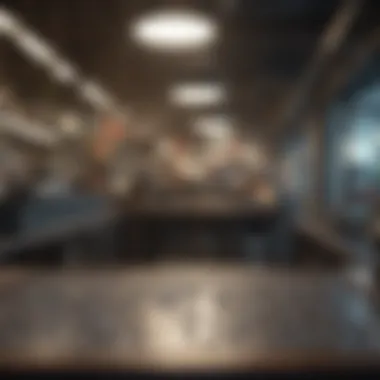
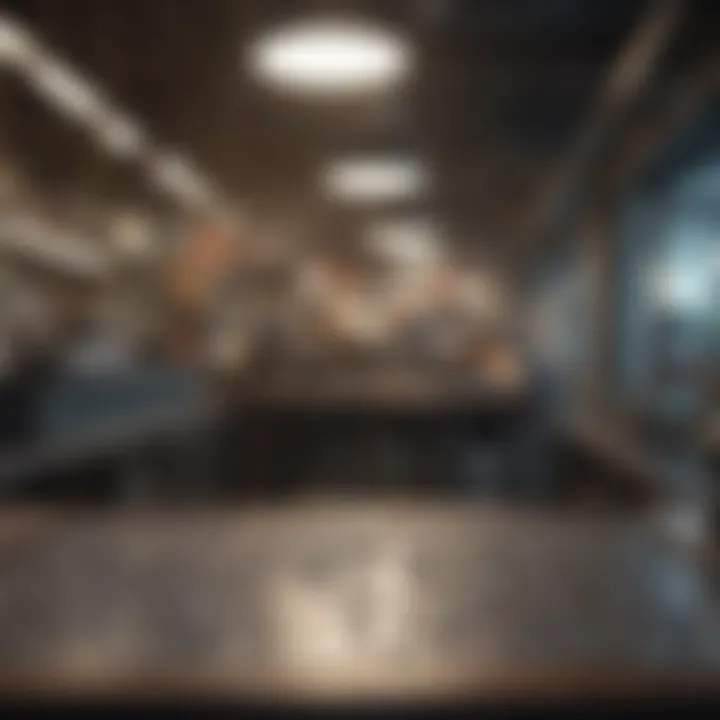
Consider a scenario in which a web application needs to handle a large volume of user requests. By using hashing tables to cache frequently accessed data, the application can minimize database queries, thus improving response times and user experience. This case exemplifies how hashing tables play a pivotal role in ensuring efficient algorithm performance in real-world applications.
Hashing Techniques in Network Security
Hashing techniques are imperative in the realm of network security. They help secure data integrity and confidentiality in various applications. In digital signatures, for instance, hashing algorithms validate the authenticity of messages. By hashing the message content and encrypting the hash, one can provide a verifiable signature without exposing the actual content.
Another case study involves the use of hashing in password storage. Instead of storing plain text passwords, systems often store hashed versions. This practice enhances security because even if a database breach occurs, attackers will confront hashed data instead of clear passwords. Techniques like bcrypt and Argon2 are popular for this purpose due to their robustness against brute-force attacks.
Moreover, hashing tables are utilized in high-performance systems for intrusion detection. Tracking user behavior and comparing it against a database of known patterns can be efficiently managed through hashing techniques. This ensures that abnormal activities are flagged swiftly, helping protect the network from threats without overburdening system resources.
In summary, case studies illustrate the various applications and benefits of hashing tables in algorithm optimization and network security. These real-world examples help to connect theoretical concepts to practical implementations, offering insights and inspiration for future innovations.
Challenges in Hashing
Hashing tables are essential in many computing applications, but they come with specific challenges that can affect their performance and efficiency. Understanding these challenges is vital for anyone engaged in computer science or related fields. The most pressing issues to consider include handling high collision rates and scalability concerns. These challenges can significantly impact the effectiveness of hashing tables, making it crucial to address them adequately.
Handling High Collision Rates
Collisions occur when two keys hash to the same index in the hash table. This scenario leads to inefficient data retrieval and increases the time complexities associated with these operations. Addressing high collision rates involves adopting rigorous strategies such as chaining and open addressing.
- Chaining: This method involves maintaining a list of all entries that hash to the same index. While it resolves collisions, it may lead to degradation of performance if the lists grow long.
- Open Addressing: In this technique, when a collision happens, the algorithm searches for the next available slot according to a predefined sequence. This approach can capitalize on the sparse nature of hash tables but can suffer from clustering issues when many entries contend for limited slots.
A high rate of collisions can lead to notable performance declines. As the load factor increases, the likelihood of collisions grows, further complicating retrieval operations. Algorithms must be tailored to balance load factors appropriately, keeping efficiency intact.
"Effective collision management is not only a matter of performance but also a fundamental principle in ensuring that hash tables serve their intended purpose efficiently."
Scalability Issues
Scalability poses a unique challenge for hashing tables. As data continues to grow exponentially, maintaining efficient operations becomes increasingly complex. Hashing tables must be designed to accommodate this growth without sacrificing performance.
Key considerations involve:
- Dynamic Resizing: Often, resizing the hash table can restore balance and reduce load factors. However, this operation can be costly in terms of time complexity due to rehashing all existing entries.
- Distributions of Inputs: A poorly chosen hash function can lead to uneven distributions, increasing collision rates and preventing the table from scaling effectively. Uniform distribution is essential for performance.
- Memory Management: Efficient memory usage becomes critical as the size of the data grows. Hash tables must be implemented with careful consideration of memory allocation to avoid excessive overhead.
By addressing scalability issues, hash tables can maintain their role as efficient data structures in diverse applications. Understanding these aspects is paramount for developers and researchers who wish to harness the full potential of hashing technologies in an ever-evolving data landscape.
Future of Hashing Technologies
The exploration of hashing technologies is vital in understanding the evolving landscape of data management and security. As organizations increasingly rely on large datasets and require efficient access patterns, innovation in hashing techniques becomes critical. The future holds numerous potential developments that can address current limitations and enhance performance. The ongoing research into hashing serves to improve the speed, security, and efficiency of data retrieval systems, ensuring they meet the demands of modern computing environments.
Emerging Trends
Several emerging trends point to the advancement of hashing technologies. Notably, the integration of machine learning with hashing techniques is gaining traction. Machine learning algorithms can optimize hash functions by training them on various datasets to produce better distribution of keys. This approach can reduce the probability of collisions and lead to more consistent performance experiences across different applications.
Additionally, as quantum computing progresses, there is a pressing need to develop quantum-resistant hashing algorithms. Existing cryptographic methods may be vulnerable to threats posed by quantum machines. Therefore, research into post-quantum hashing will be pivotal in securing data in the future, allowing organizations to safeguard sensitive information against potential breaches.
- Machine learning optimization
- Quantum-resistant algorithms
- Integration with blockchain for enhanced security
Innovations in Hashing Algorithms
Innovative hashing algorithms are emerging to address performance and security drawbacks of existing techniques. For example, the development of adaptive hashing is one such innovation. This technique adjusts its hashing strategy based on the characteristics of incoming data, enhancing efficiency during peak load times. Furthermore, dynamic hash tables that resize automatically offer flexibility, saving memory and processing power without sacrificing speed.
Also, advances in the use of salted hashes provide better security in storing passwords and sensitive data. Salting adds unique data to each entry before hashing, making it more challenging for attackers to use precomputed hash tables in cracking passwords. This improves overall security efficacy.
In summary, the future of hashing technologies appears promising, with various trends and innovations paving the way for more efficient, secure, and robust data management solutions. Continued exploration and implementation of these advancements will certainly impact not only academic research but also practical applications in different sectors.
"The consolidation of hashing technologies with modern computational advancements points toward a future where data access becomes not only faster but secure against emerging threats."
By staying abreast of these trends and innovations, professionals can leverage hashing technologies more effectively, ensuring they remain at the forefront of their fields.
Culmination
In this article, we have explored hashing tables, uncovering their critical role in data structures and algorithms. The concluding thoughts surrounding this topic emphasize not only the practical utility of hashing tables but also their theoretical significance.
Summary of Key Points
The key points of the article illustrate the importance of hashing tables in various applications. Key highlights include:
- Fundamental principles: Hash tables store data using hash functions to compute an index into an array of buckets or slots. This leads to efficient data retrieval.
- Collision handling: Techniques like chaining and open addressing provide effective means to manage instances where multiple keys hash to the same index.
- Performance metrics: Time complexity and space complexity are vital in evaluating hashing efficacy, offering insight into performance under different conditions.
- Applications: From database management to data integrity verification, the versatile usage of hashing tables cannot be ignored.
"Hash tables are a pivotal mechanism for optimizing look-up times, essential in modern computing environments."
Implications for Future Research
The future of hashing technology presents abundant opportunities for research and advancement. Potential areas for exploration include:
- Emerging trends in algorithms: Investigating next-generation hashing functions, which might improve performance and security.
- Scalability solutions: As data grows, optimizing hash tables for larger datasets while maintaining efficiency remains crucial.
- Real-world applications: More case studies could help identify unique use cases across various sectors, enhancing our understanding of hash table implementation.
In summary, the significance of hashing tables extends far beyond academic interest, making them relevant in a world increasingly reliant on rapid data processing and retrieval. As we advance in technology, continuing to explore and refine hash table strategies will be essential.